Important
webext-bridge
just joined the Server Side Up family of open source projects. Read the announcement โ
Messaging in web extensions made easy. Batteries included. Reduce headache and simplify the effort of keeping data in sync across different parts of your extension. webext-bridge
is a tiny library that provides a simple and consistent API for sending and receiving messages between different parts of your web extension, such as background
, content-script
, devtools
, popup
, options
, and window
contexts.
// Inside devtools script
import { sendMessage } from "webext-bridge/devtools";
button.addEventListener("click", async () => {
const res = await sendMessage(
"get-selection",
{ ignoreCasing: true },
"content-script"
);
console.log(res); // > "The brown fox is alive and well"
});
// Inside content script
import { sendMessage, onMessage } from "webext-bridge/content-script";
onMessage("get-selection", async (message) => {
const {
sender,
data: { ignoreCasing },
} = message;
console.log(sender.context, sender.tabId); // > devtools 156
const { selection } = await sendMessage(
"get-preferences",
{ sync: false },
"background"
);
return calculateSelection(data.ignoreCasing, selection);
});
// Inside background script
import { onMessage } from "webext-bridge/background";
onMessage("get-preferences", ({ data }) => {
const { sync } = data;
return loadUserPreferences(sync);
});
Examples above require transpilation and/or bundling using
webpack
/babel
/rollup
webext-bridge
handles everything for you as efficiently as possible. No more chrome.runtime.sendMessage
or chrome.runtime.onConnect
or chrome.runtime.connect
....
We put together a comprehensive guide to help people build multi-platform browser extensions. The book covers everything from getting started to advanced topics like messaging, storage, and debugging. It's a great resource for anyone looking to build a browser extension. The book specifically covers how to use webext-bridge
to simplify messaging in your extension.
$ npm i webext-bridge
Just import { } from 'webext-bridge/{context}'
wherever you need it and use as shown in example above
Even if your extension doesn't need a background page or wont be sending/receiving messages in background script.
webext-bridge
uses background/event context as staging area for messages, therefore it must loaded in background/event page for it to work.
(Attempting to send message from any context will fail silently ifwebext-bridge
isn't available in background page).
See troubleshooting section for more.
As we are likely to use sendMessage
and onMessage
in different contexts, keeping the type consistent could be hard, and its easy to make mistakes. webext-bridge
provide a smarter way to make the type for protocols much easier.
Create shim.d.ts
file with the following content and make sure it's been included in tsconfig.json
.
// shim.d.ts
import { ProtocolWithReturn } from "webext-bridge";
declare module "webext-bridge" {
export interface ProtocolMap {
foo: { title: string };
// to specify the return type of the message,
// use the `ProtocolWithReturn` type wrapper
bar: ProtocolWithReturn<CustomDataType, CustomReturnType>;
}
}
import { onMessage } from 'webext-bridge/content-script'
onMessage('foo', ({ data }) => {
// type of `data` will be `{ title: string }`
console.log(data.title)
}
import { sendMessage } from "webext-bridge/background";
const returnData = await sendMessage("bar", {
/* ... */
});
// type of `returnData` will be `CustomReturnType` as specified
Sends a message to some other part of your extension.
Notes:
-
If there is no listener on the other side an error will be thrown where
sendMessage
was called. -
Listener on the other may want to reply. Get the reply by
await
ing the returnedPromise
-
An error thrown in listener callback (in the destination context) will behave as usual, that is, bubble up, but the same error will also be thrown where
sendMessage
was called -
If the listener receives the message but the destination disconnects (tab closure for exmaple) before responding,
sendMessage
will throw an error in the sender context.
Required |
string
Any string
that both sides of your extension agree on. Could be get-flag-count
or getFlagCount
, as long as it's same on receiver's onMessage
listener.
Required |
any
Any serializable value you want to pass to other side, latter can access this value by refering to data
property of first argument to onMessage
callback function.
Required |
string |
The actual identifier of other endpoint.
Example: devtools
or content-script
or background
or content-script@133
or devtools@453
content-script
, window
and devtools
destinations can be suffixed with @<tabId>
to target specific tab. Example: devtools@351
, points to devtools panel inspecting tab with id 351.
For content-script
, a specific frameId
can be specified by appending the frameId
to the suffix @<tabId>.<frameId>
.
Read Behavior
section to see how destinations (or endpoints) are treated.
Note: For security reasons, if you want to receive or send messages to or from
window
context, one of your extension's content script must callallowWindowMessaging(<namespace: string>)
to unlock message routing. Also callsetNamespace(<namespace: string>)
in thosewindow
contexts. Use same namespace string in those two calls, sowebext-bridge
knows which message belongs to which extension (in case multiple extensions are usingwebext-bridge
in one page)
Register one and only one listener, per messageId per context. That will be called upon sendMessage
from other side.
Optionally, send a response to sender by returning any value or if async a Promise
.
Required |
string
Any string
that both sides of your extension agree on. Could be get-flag-count
or getFlagCount
, as long as it's same in sender's sendMessage
call.
Required |
fn
A callback function Bridge
should call when a message is received with same messageId
. The callback function will be called with one argument, a BridgeMessage
which has sender
, data
and timestamp
as its properties.
Optionally, this callback can return a value or a Promise
, resolved value will sent as reply to sender.
Read security note before using this.
Caution: Dangerous action
API available only to content scripts
Unlocks the transmission of messages to and from window
(top frame of loaded page) contexts in the tab where it is called.
webext-bridge
by default won't transmit any payload to or from window
contexts for security reasons.
This method can be called from a content script (in top frame of tab), which opens a gateway for messages.
Once again, window
= the top frame of any tab. That means allowing window messaging without checking origin first will let JavaScript loaded at https://evil.com
talk with your extension and possibly give indirect access to things you won't want to, like history
API. You're expected to ensure the
safety and privacy of your extension's users.
Required |
string
Can be a domain name reversed like com.github.facebook.react_devtools
or any uuid
. Call setNamespace
in window
context with same value, so that webext-bridge
knows which payload belongs to which extension (in case there are other extensions using webext-bridge
in a tab). Make sure namespace string is unique enough to ensure no collisions happen.
API available to scripts in top frame of loaded remote page
Sets the namespace Bridge
should use when relaying messages to and from window
context. In a sense, it connects the callee context to the extension which called allowWindowMessaging(<namespace: string>)
in it's content script with same namespace.
Required |
string
Can be a domain name reversed like com.github.facebook.react_devtools
or any uuid
. Call setNamespace
in window
context with same value, so that webext-bridge
knows which payload belongs to which extension (in case there are other extensions using webext-bridge
in a tab). Make sure namespace string is unique enough to ensure no collisions happen.
The following API is built on top of sendMessage
and onMessage
, basically, it's just a wrapper, the routing and security rules still apply the same way.
Opens a Stream
between caller and destination.
Returns a Promise
which resolves with Stream
when the destination is ready (loaded and onOpenStreamChannel
callback registered).
Example below illustrates a use case for Stream
Required |
string
Stream
(s) are strictly scoped sendMessage
(s). Scopes could be different features of your extension that need to talk to the other side, and those scopes are named using a channel id.
Required |
string
Same as destination
in sendMessage(msgId, data, destination)
Registers a listener for when a Stream
opens.
Only one listener per channel per context
Required |
string
Stream
(s) are strictly scoped sendMessage
(s). Scopes could be different features of your extension that need to talk to the other side, and those scopes are named using a channel id.
Required |
fn
Callback that should be called whenever Stream
is opened from the other side. Callback will be called with one argument, the Stream
object, documented below.
Stream
(s) can be opened by a malicious webpage(s) if your extension's content script in that tab has called allowWindowMessaging
, if working with sensitive information use isInternalEndpoint(stream.info.endpoint)
to check, if false
call stream.close()
immediately.
// background.js
// To-Do
Following rules apply to
destination
being specified insendMessage(msgId, data, destination)
andopenStream(channelId, initialData, destination)
-
Specifying
devtools
as destination fromcontent-script
will auto-route payload to inspectingdevtools
page if open and listening. If devtools are not open, message will be queued up and delivered when devtools are opened and the user switches to your extension's devtools panel. -
Specifying
content-script
as destination fromdevtools
will auto-route the message to inspected window's topcontent-script
page if listening. If page is loading, message will be queued up and delivered when page is ready and listening. -
If
window
context (which could be a script injected by content script) are source or destination of any payload, transmission must be first unlocked by callingallowWindowMessaging(<namespace: string>)
inside that page's top content script, sinceBridge
will first deliver the payload tocontent-script
using rules above, and latter will take over and forward accordingly.content-script
<->window
messaging happens usingwindow.postMessage
API. Therefore to avoid conflicts,Bridge
requires you to callsetNamespace(uuidOrReverseDomain)
inside the said window script (injected or remote, doesn't matter). -
Specifying
devtools
orcontent-script
orwindow
frombackground
will throw an error. When calling frombackground
, destination must be suffixed with tab id. Likedevtools@745
fordevtools
inspecting tab id 745 orcontent-script@351
for topcontent-script
at tab id 351.
The following note only applies if and only if, you will be sending/receiving messages to/from window
contexts. There's no security concern if you will be only working with content-script
, background
, popup
, options
, or devtools
scope, which is the default setting.
window
context(s) in tab A
get unlocked the moment you call allowWindowMessaging(namespace)
somewhere in your extension's content script(s) that's also loaded in tab A
.
Unlike chrome.runtime.sendMessage
and chrome.runtime.connect
, which requires extension's manifest to specify sites allowed to talk with the extension, webext-bridge
has no such measure by design, which means any webpage whether you intended or not, can do sendMessage(msgId, data, 'background')
or something similar that produces same effect, as long as it uses same protocol used by webext-bridge
and namespace set to same as yours.
So to be safe, if you will be interacting with window
contexts, treat webext-bridge
as you would treat window.postMessage
API.
Before you call allowWindowMessaging
, check if that page's window.location.origin
is something you expect already.
As an example if you plan on having something critical, always verify the sender
before responding:
// background.js
import { onMessage, isInternalEndpoint } from "webext-bridge/background";
onMessage("getUserBrowsingHistory", (message) => {
const { data, sender } = message;
// Respond only if request is from 'devtools', 'content-script', 'popup', 'options', or 'background' endpoint
if (isInternalEndpoint(sender)) {
const { range } = data;
return getHistory(range);
}
});
- Doesn't work?
Ifwindow
contexts are not part of the puzzle,webext-bridge
works out of the box for messaging betweendevtools
<->background
<->content-script
(s). If even that is not working, it's likely thatwebext-bridge
hasn't been loaded in background page of your extension, which is used bywebext-bridge
as a relay. If you don't need a background page for yourself, here's bare minimum to getwebext-bridge
going.
// background.js (requires transpiration/bundling using webpack(recommended))
import "webext-bridge/background";
// manifest.json
{
"background": {
"scripts": ["path/to/transpiled/background.js"]
}
}
- Can't send messages to
window
?
Sending or receiving messages from or towindow
requires you to open the messaging gateway in content script(s) for that particular tab. CallallowWindowMessaging(<namespaceA: string>)
in any of your content script(s) in that tab and callsetNamespace(<namespaceB: string>)
in the script loaded in top frame i.e thewindow
context. Make sure thatnamespaceA === namespaceB
. If you're doing this, read the security note above
- Discord for friendly support from the community and the team.
- GitHub for source code, bug reports, and project management.
- Get Professional Help - Get video + screen-sharing help directly from the core contributors.
As an open-source project, we strive for transparency and collaboration in our development process. We greatly appreciate any contributions members of our community can provide. Whether you're fixing bugs, proposing features, improving documentation, or spreading awareness - your involvement strengthens the project. Please review our contribution guidelines and code of conduct to understand how we work together respectfully.
- Bug Report: If you're experiencing an issue while using these images, please create an issue.
- Feature Request: Make this project better by submitting a feature request.
- Documentation: Improve our documentation by submitting a documentation change.
- Community Support: Help others on GitHub Discussions or Discord.
- Security Report: Report critical security issues via our responsible disclosure policy.
Need help getting started? Join our Discord community and we'll help you out!
All of our software is free an open to the world. None of this can be brought to you without the financial backing of our sponsors.
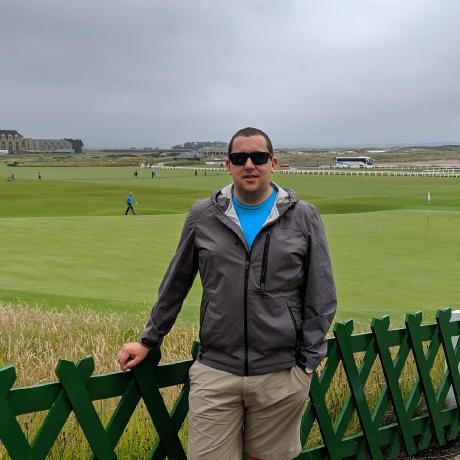

We're Dan and Jay - a two person team with a passion for open source products. We created Server Side Up to help share what we learn.
- ๐ Blog - Get the latest guides and free courses on all things web/mobile development.
- ๐ Community - Get friendly help from our community members.
- ๐คตโโ๏ธ Get Professional Help - Get video + screen-sharing support from the core contributors.
- ๐ป GitHub - Check out our other open source projects.
- ๐ซ Newsletter - Skip the algorithms and get quality content right to your inbox.
- ๐ฅ Twitter - You can also follow Dan and Jay.
- โค๏ธ Sponsor Us - Please consider sponsoring us so we can create more helpful resources.
If you appreciate this project, be sure to check out our other projects.
- The Ultimate Guide to Building APIs & SPAs: Build web & mobile apps from the same codebase.
- Building Multi-Platform Browser Extensions: Ship extensions to all browsers from the same codebase.
- Bugflow: Get visual bug reports directly in GitHub, GitLab, and more.
- SelfHost Pro: Connect Stripe or Lemonsqueezy to a private docker registry for self-hosted apps.
- serversideup/php Docker Images: PHP Docker images optimized for Laravel and running PHP applications in production.
- Financial Freedom: Open source alternative to Mint, YNAB, & Monarch Money.
- AmplitudeJS: Open-source HTML5 & JavaScript Web Audio Library.
- webext-bridge: Messaging in web extensions made easy. Batteries included.