Update: Requestly's Android Debugger has been sunset. This repository has been archived.
Requestly Android SDK lets you debug your android apps without needing you to setup any proxies or install any certificates everytime. It makes easy to identify & debug your Android Apps faster and save your time.
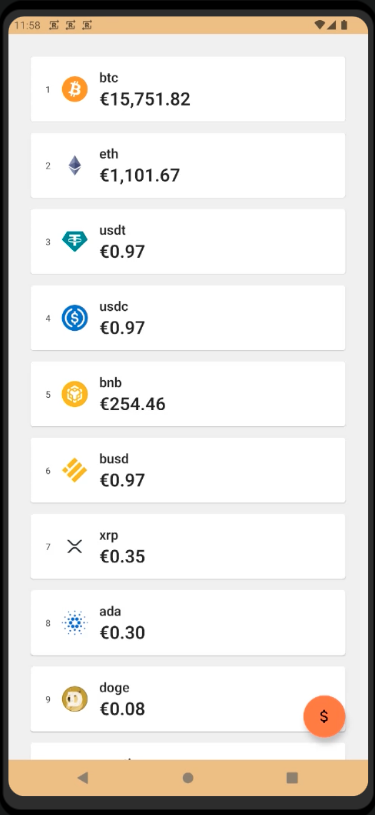
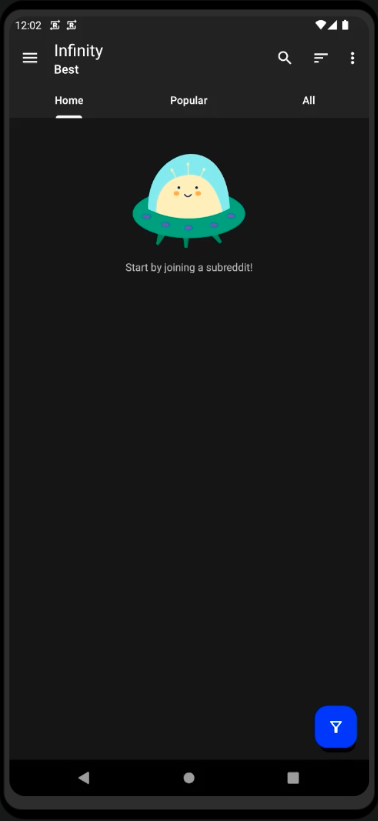
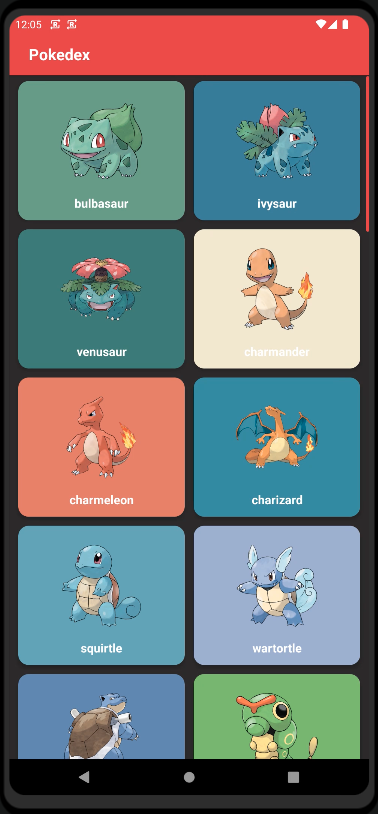
The best way to install the Requestly Android SDK is with a build system like Gradle. This ensures you can easily upgrade to the latest versions.
RQInterceptor is distributed through Maven Central. To use it you need to add the following Gradle dependency to your build.gradle file of you android app module (NOT the root file)
dependencies {
debugImplementation "io.requestly:requestly-android:2.4.9"
releaseImplementation "io.requestly:requestly-android-noop:2.4.9"
debugImplementation "io.requestly:requestly-android-okhttp:2.4.9"
releaseImplementation "io.requestly:requestly-android-okhttp-noop:2.4.9"
}
Initialize the Requestly SDK in your Application class onCreate method.
class App : Application(){
override fun onCreate() {
super.onCreate()
// Initialize Requestly SDK like this
Requestly.Builder(this, [optional "<your-sdk-key>"])
.build()
}
}
sdk-key
is optional. You can use local devtool features without sdk-key.
To get the sdk key, you need to create an app. Follow the steps here to create an app.
To configure the Interceptor, you need to initialize the RQCollector and then add rqInterceptor as the last interceptor to your okHttpClient
val collector = RQCollector(context=appContext)
val rqInterceptor = RQInterceptor.Builder(appContext)
.collector(collector)
.build()
val client = OkHttpClient.Builder()
.addInterceptor(rqInterceptor)
.build()
Retrofit.Builder()
.baseUrl(APIUtils.API_BASE_URI)
.client(okHttpClient) // okHttpClient with RQInterceptor
.build();
Lets you view and modify HTTP traffic. It comes with these capabilities to:
- InApp Inspector : Directly view your HTTP request from your phone.
- Modify API: Modify Response, Redirect Request, Delay Request and many more.
Debug & Validate your Analytics Events directly from your App. The SDK provides a simple API to send your events.
RequestlyEvent.send(<eventName: String>, <eventData:Map<String, Any>>)
Debug your Logs directly from your App. No need to connect your device to your computer to know what's happening inside your app.
Switch between production and staging APIs easily in your Android debug builds. Eg. api.requestly.io → staging.requestly.io
Special Thanks to chuckerteam for maintaining such an awesome project Chucker because of which requestly-android-sdk was possible.