Hey there,
I upgradet to Pedantic 1.20 but came across a severe side effect error in pedantic.
All of my ~130 tests were successful in version<1.20 but now in 1.20 there are 4 failing tests.
All of them refer to this function of my stack-class:
def top(self) -> Optional[T]:
if len(self.items) > 0:
return self.items[len(self.items)-1]
else:
return None
When the stack is empty, I want to return None, which I typehint with Optional.
Sometimes pedantic accepts this function and sometimes not! It depends on the order of my unit tests... Look at the two pictures:
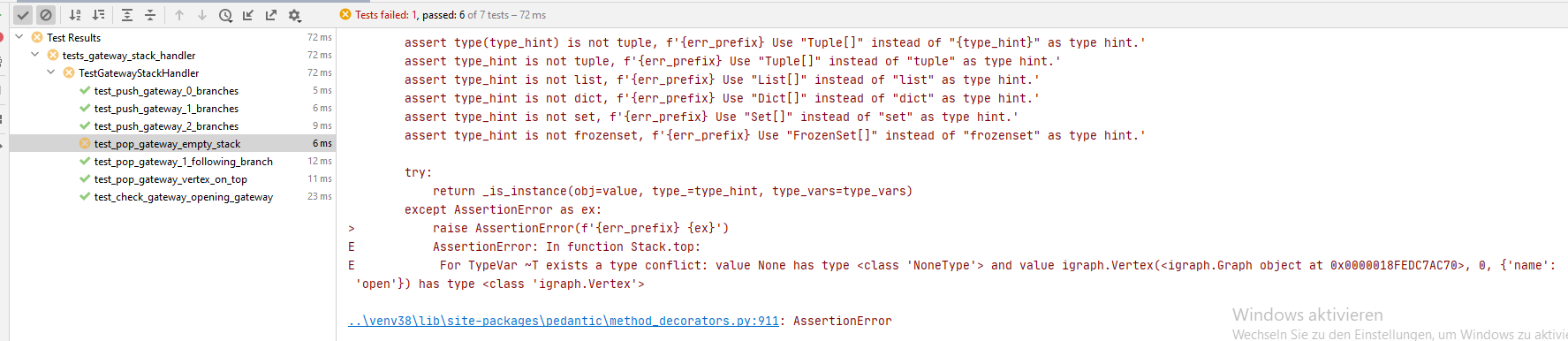
If I put the failed test at first, other tests fail:
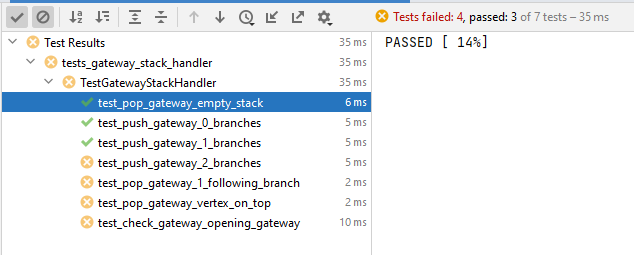
If I execute my test solely, everything is fine.
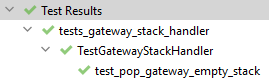
All of my tests are independent from each other. If I follow the debugger, sometimes pedantic throws an error and sometimes not - with everything else being equal.
Here is the full implementation of my stack class:
from typing import List, Optional, Union
from typing import TypeVar, Generic
from pedantic import pedantic_class
T = TypeVar('T')
@pedantic_class
class Stack(Generic[T]):
def __init__(self) -> None:
self.items: List[T] = []
def push(self, item: T) -> None:
self.items.append(item)
def pop(self) -> T:
return self.items.pop()
def empty(self) -> bool:
return not self.items
def top(self) -> Optional[T]:
if len(self.items) > 0:
return self.items[len(self.items)-1]
else:
return None
So far, in all of every tests I instantiate a new stack object with an empty list of items. And I only put igraph.Vertex objects in it.
......
I spend some time in the debugger understanding this behaviour:
The first time I call stack.top() and let it return a None, everything is fine.
Second, third and n-th time returning a None will be fine.
But then the first time calling stack.top() when expecting to return a igraph.Vertex(), pedantic screams at me:
AssertionError: In function Stack.top:
E For TypeVar ~T exists a type conflict: value None has type <class 'NoneType'> and value igraph.Vertex(<igraph.Graph object at 0x000001DDB10FBC70>, 0, {'name': 'open'}) has type <class 'igraph.Vertex'>
Pedantic expects a None.
So it looks like, your inner pedantic workings bind 'T' only to a None and not to None and the runtime-T-type.
Hope you can fix it.