TOP 4 RISING AND TRENDING CLOUD PROVIDERS AND THEIR FULL INFORMATION WITH THEIR LINKS TO SEARCH ON-
SO HERE 1st comes -
AMAZON WEB SERVICES
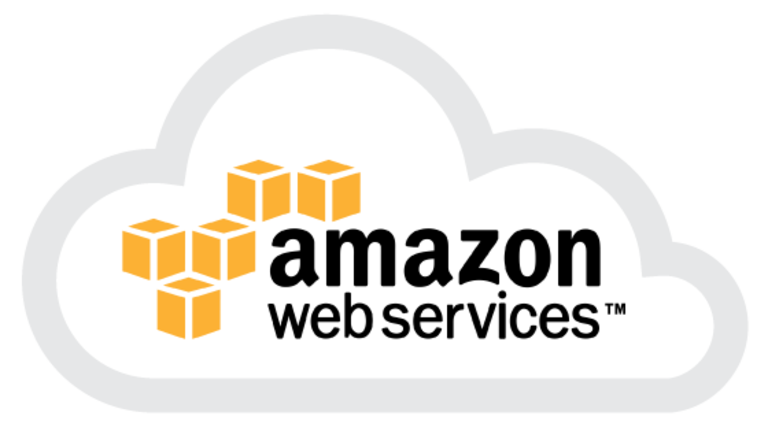
Amazon Web Service provides cloud computing services, including IaaS and PaaS, and is widely considered the leading cloud vendor.
By all accounts, Amazon Web Services (AWS) is the world's largest public cloud computing service. And although several other cloud computing providers are currently growing more quickly than Amazon, John Dinsdale, a chief analyst and research director at Synergy Research Group, said that Amazon remains "in a field of its own."
According to the company website, AWS currently operates 42 different "availability zones," which it defines as "one or more discrete data centers, each with redundant power, networking and connectivity, housed in separate facilities." It currently has data centers in 16 different geographic regions, and it plans to expand to three more regions before the end of 2017.
Amazon Web Services (AWS) is the undisputed market leader in cloud computing. According to Amazon's most recent quarterly financial report, AWS generated $2.9 billion in revenue for the quarter ending June 30, 2016, up from $1.8 billion during the same quarter last year.
The company offers a complete range of IaaS and PaaS services. Among the best known are its Elastic Compute Cloud (EC2), Elastic Beanstalk, Simple Storage Service (S3), Elastic Block Store (EBS), Glacier storage, Relational Database Service (RDS), and DynamoDB NoSQL database. It also offers cloud services related to networking, analytics and machine learning, the Internet of Things (IoT), mobile services, development, cloud management, cloud security and more.
LINK TO SEARCH ON DEPTH ABOUT IT-https://aws.amazon.com/
AWS Services
Amazon divides its extensive portfolio of cloud computing services into 19 different categories:
1.Compute — includes its best-known product, EC2, as well as its Container Service, Virtual Private 2.Cloud, Elastic Beanstalk and the Lambda serverless computing service, among others
3.Storage — includes S3, as well as Elastic Block Storage, Glacier, Snowball and others
4.Database — includes both relational and NoSQL databases, including Aurora, Amazon RDS, 5.DynamoDB, ElastiCache, Redshift and more
6.Migration — includes services to help enterprises move from traditional data centers to the public cloud
7.Networking and Content Delivery — includes Elastic Load Balancing, the Route 53 DNS service, the CloudFront content delivery network and more
8.Developer Tools — includes multiple tools to support DevOps and Agile software development, including CodeCommit repositories, CodePipeline continuous integration and delivery, CodeBuild testing, CodeDeploy deployment automation, etc.
9.Management Tools — includes services to help administrators monitor and manage hybrid cloud infrastructure
10.Artificial Intelligence — includes Lex chatbot services, Polly text-to-speech, Rekognition image analysis and the Amazon Machine Learning platform
Analytics — includes big data tools like the EMR Hadoop framework, Kinesis streaming data, Glue ETL, QuickSight business intelligence and more
Security, Identity and Compliance — includes cloud security tools such as Identity and Access 11.Management (IAM), Inspector security assessment, Shield DDOS Protection and many other services
12.Mobile Services — includes mobile development tools like the Mobile Hub and the Mobile SDK
13.Application Services — includes Step Functions, API Gateway and Elastic Transcoder
14.Messaging — includes the SQS, Simple Notification Service (SNS), Pinpoint push notifications and Simple Email Service (SES)
15.Business Productivity — includes Chime communications services, WorkDocs enterprise storage and sharing service and WorkMail secure email
16.Desktop and App Streaming — includes the Workspaces desktop-as-a-service offering and AppStream, which streams desktop applications to a browser
17.Software — includes third-party software as a service available through the AWS Marketplace
Internet of Things — includes the AWS IoT Platform, Greeengrass and IoT Button programmable devices
18.Contact Center — includes Amazon's cloud-based, self-service call center, called Amazon Connect
19.Game Development — includes GameLift game server hosting and the free Lumberyard 3D game engine.
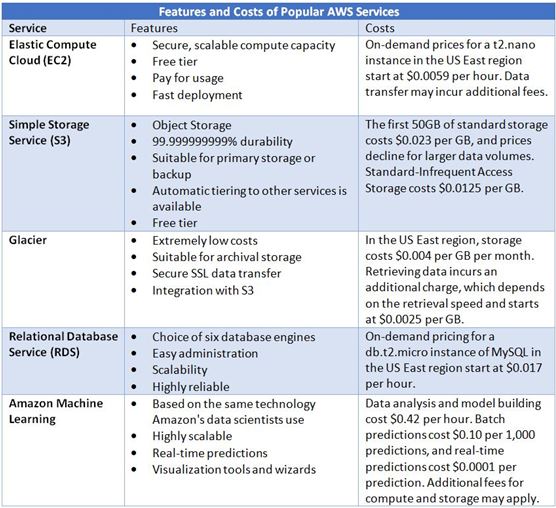
WHY WE SWITH TO AMAZON WEB SERVICES(AWS)-
In comparison with the other major public cloud vendors — Microsoft Azure, Google Cloud Platform and IBM — AWS has a few clear advantages.
First is its sheer size. Amazon's list of services has a breadth and depth that few other public cloud providers can match. It has data centers spread all around the world, and it has a long and growing list of customers. That's part of the reason why Gartner concluded, "Although AWS will not be the ideal fit for every need, it has become the 'safe choice' in this market, appealing to customers who desire the broadest range of capabilities and long-term market leadership."
Second, Amazon's prices are generally comparable to the other major cloud vendors for most use cases. Economies of scale have given the company the ability to drop its prices repeatedly, and it continues to do so on a regular basis.
Third, AWS is very popular with developers. That's not surprising, given that it was born out of Amazon's need to simplify IT infrastructure for its own developers. Startup developers who use AWS often find that it is simplest to keep using the cloud service as their companies grow. And enterprise developers who like to use AWS for dev and test are often influential is selecting the service for production workloads as well.
SECOND COMES-
MICROSOFT AZURE-
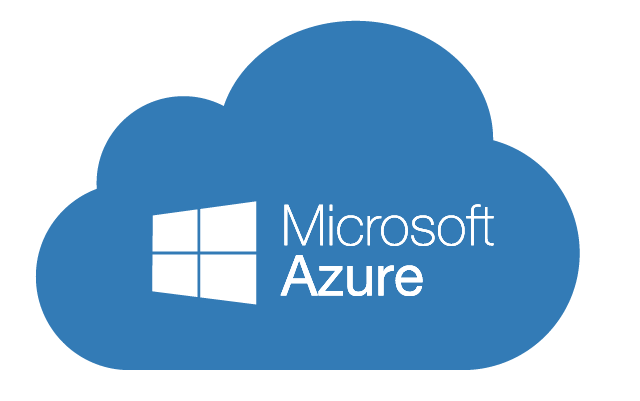
It's a little more difficult to figure out how much revenue Microsoft generates from cloud computing. In its fiscal 2016 Q4 report, the company said that its "Intelligent Cloud" revenue increased 5 percent to reach $6.7 billion. That makes it seem like Microsoft does more cloud business than Amazon, except that Microsoft includes its very substantial server revenues along with its Azure cloud computing service in the Intelligent Cloud category. The company has previously said that its Azure business is on a $10 billion annual run rate, or around $2.5 billion in revenue per quarter. Most market analysts put it in the number two spot behind Amazon.
In addition to its Azure IaaS and PaaS offerings, Microsoft also has several SaaS offerings, including its Office 365 products, the online versions of its Dynamics line of enterprise software and its online developer tools.
For organizations looking for a public cloud provider, Microsoft stands out as one of the top vendors in the market with a wide range of services available.
Microsoft offers all three of the major categories of cloud computing: software as a service (SaaS), infrastructure as a service (IaaS) and platform as a service (PaaS). It sells its IaaS and PaaS offerings under the Microsoft Azure brand name.
By nearly all estimates, Azure is the second largest IaaS and PaaS service on the planet, trailing behind Amazon Web Services (AWS). It is particularly popular among large, geographically dispersed enterprises, especially those that use Microsoft software like Windows and Office.
Today, Microsoft Azure enjoys a reputation as a mature, reliable and highly secure public cloud provider. However, Azure has had to play a lot of catch up to earn this spot.
Microsoft Azure Services
Microsoft Azure offers an extremely large portfolio of cloud services, which it divides into Thirteen categories:
1.Compute — includes Virtual Machines, Virtual Machine Scale Sets, Functions for serverless computing, Batch for containerized batch workloads, Service Fabric for microservices and container orchestration, and Cloud Services for building cloud-based apps and APIs
2.Networking — includes a variety of networking tools, like the Virtual Network, which can connect to on-premise data centers; Load Balancer; Application Gateway; VPN Gateway; Azure DNS for domain hosting, Content Delivery Network, Traffic Manager, ExpressRoute dedicated private network fiber connections; and Network Watcher monitoring and diagnostics
3.Storage — includes Blob, Queue, File and Disk Storage, as well as a Data Lake Store, Backup and Site Recovery, among others
4.Web + Mobile — includes several services for building and deploying applications, but the most notable is probably the App Service, which comprises services for Web Apps, Mobile Apps, Logic Apps (a low-code, data-driven service) and API Apps (for creating and using APIs)
5.Containers — includes Container Service, which supports Kubernetes, DC/OS or Docker Swarm, and Container Registry, as well as tools for microservices
6.Databases — includes several SQL-based databases and related tools, as well as Cosmos DB, Table Storage for NoSQL and Redis Cache in-memory technology
7.Data + Analytics — includes big data tools like HDInsight for Hadoop Spark, R Server, HBase and Storm clusters; Stream Analytics; Data Lake Analytics; and Power BI Embedded, among others
8.AI + Cognitive Services — includes multiple tools for developing applications with artificial intelligence capabilities, like the Computer Vision API, Face API, Bing Web Search, Video Indexer, Language Understanding Intelligent Service and more
9.Internet of Things — includes IoT Hub and IoT Edge services that can be combined with a variety of machine learning, analytics and communications services
Enterprise Integration — includes multiple tools for building and managing hybrid cloud computing environments
10.Security + Identity — includes Security Center, Azure Active Directory, Key Vault and Multi-Factor Authentication Services
11.Developer Tools — includes cloud development services like Visual Studio Team Services, Azure DevTest Labs, HockeyApp mobile app deployment and monitoring, Xamarin cross-platform mobile development and more
12.Monitoring + Management — includes numerous tools for managing Azure workloads and hybrid cloud environments, such as the Microsoft Azure Port, Azure Resource Manager, Log Analytics, Automation, Scheduler and more
13.Microsoft Azure Stack — includes solutions for replicating Azure infrastructure in enterprise data centers with the goal of facilitating hybrid cloud deployments
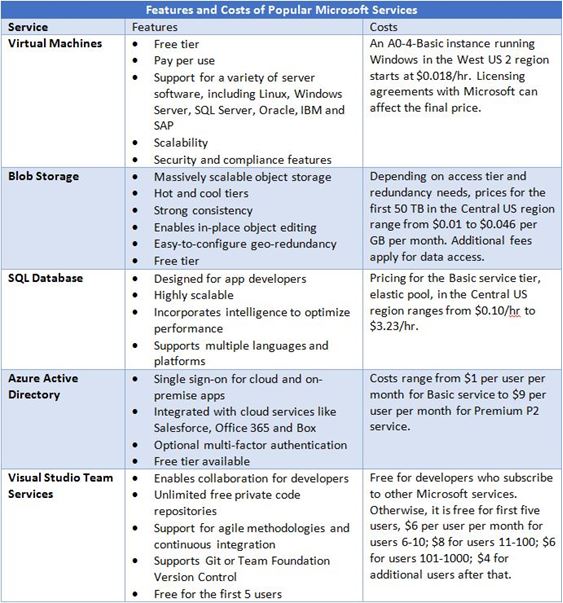
WHY YOU CHOOSE TO MICROSOFT AZURE-
The biggest advantage that Microsoft has when it comes to its cloud computing services can be summed up in one word: Windows.
For organizations that already use Microsoft software, such as Windows, Office, SQL Server, SharePoint, Dynamics, etc., using the Microsoft cloud computing service seems like a natural fit. It allows them to use the tools and interfaces that they are already familiar with and helps them get up and running quickly.
In addition, Microsoft has made a particular effort at enabling hybrid computing, and its Azure Stack solution, which is still in technical preview, seems likely to enhance its appeal for enterprises with hybrid environments.
Microsoft has also very deliberately targeted the government market. It touts its security and compliance capabilities, and its website claims, "Azure has been recognized as the most trusted cloud for U.S. government institutions, including a FedRAMP High authorization that covers 18 Azure services."
Finally, Microsoft Azure has a very vibrant ecosystem with a lot of partnerships with other technology vendors. For example, it has relationships with Red Hat, Canonical, Citrix, HPE, Adobe, SAP, Cisco and many others, that enhance the services it is able to offer customers.
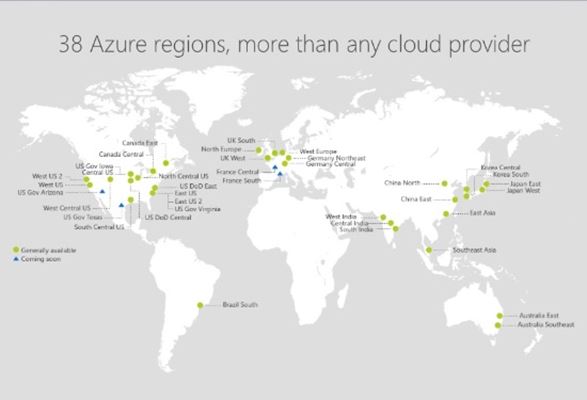
Microsoft’s map of the Azure regions, which span the globe; proximity to a data center is important for cloud customers.
LINK TO GO INTO DEPTH AND SEARCH It-https://azure.microsoft.com/en-in/
THIRD COMES-
IBM CLOUD-
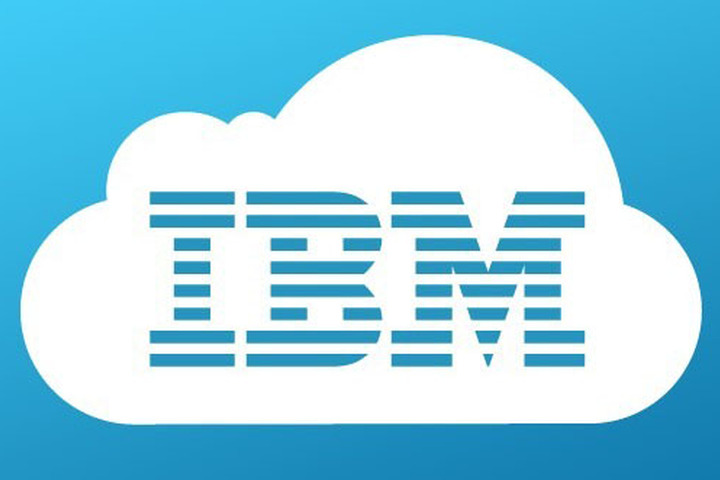
Although it hasn't always been considered one of the "big three" cloud computing vendors, IBM's cloud business has been coming on strong. In its most recent quarterly report, IBM said that its "cloud-as-a-service revenue was up 50 percent," and had an annual run rate of $6.7 billion.
IBM's most visible cloud service is its Bluemix PaaS, which is aimed primarily at enterprise development teams. The company also a lot of enterprise software on a SaaS basis, and it sells cloud infrastructure, cloud management tools and cloud managed services.
IBM's Bluemix cloud computing platform stands out for its bare metal servers, GPU servers, big data analytics and cognitive computing capabilities, as well as its developer-friendly features.
When it comes to public cloud computing vendors, IBM doesn't always enjoy the same mindshare as Amazon Web Services (AWS), Microsoft Azure and Google Cloud Platform. However, some analyst reports have claimed that IBM actually has a larger share of the infrastructure as a service (IaaS) and platform as a service (PaaS) market than Google. Other analyses place it solidly in fourth place behind the "big three." Either way, IBM is one of the largest cloud computing providers on the planet.
Telling the story of IBM's public cloud computing capabilities is complicated by the fact that IBM uses a lot of different brand names for its cloud services. The "IBM Cloud" label is an umbrella category that encompasses its hardware, software and services for helping enterprises build private clouds, as well as its Bluemix public cloud services. The "Bluemix" name used to be reserved for IBM's PaaS services for developers, but now Bluemix also offers some IaaS services.
In addition, IBM has another IaaS service called SoftLayer. Organizations can still purchase cloud computing services under the SoftLayer brand name, but IBM seems to migrating toward the Bluemix brand. These days the company describes SoftLayer as the infrastructure that forms "the core of IBM Bluemix," and the "about us" link on the SoftLayer website takes you to the Bluemix site.
If all that weren't confusing enough, over the years IBM has used a number of other brand names for its cloud services, including Cloudburst, Smart Business and SmartCloud.
IBM Cloud Services
Because IBM seems to be focusing more attention on Bluemix than SoftLayer, this list will cover its Bluemix offerings rather than those available through SoftLayer. The company divides its Bluemix Services into twelve categories:
1.Compute Infrastructure — includes its bare metal servers (single-tenant servers that are highly customizable), virtual servers, GPU computing, POWER servers (based on IBM's POWER architecture) and server software
2.Compute Services — includes OpenWhisk serverless computing, containers and Cloud Foundry runtimes
3.Storage — includes object, block and file storage, as well as server-backup capabilities
4.Network — includes load balancing, Direct Link private secure connections, network appliances, content delivery network and domain services
5.Mobile — includes IBM's Swift tools for creating iOS apps, its MobileFirst Starter package for getting a mobile app up and running, and its Mobile Foundation app back-end services
6.Watson — includes IBM's artificial intelligence and machine learning services, which it calls "cognitive computing," such as Discovery search and content analytics, Conversation natural language services and speech-to-text
7.Data and analytics — includes data services, analytics services, big data hosting, Cloudera hosting, MongoDB hosting and Riak hosting
8.Internet of Things — includes IBM's IoT platform and its IoT starter packages
9.Security — includes tools for securing cloud environments, such as a firewall, hardware security modules (physical devices with key management capabilities), Intel Trusted Execution Technology, security software and SSL certificates
10.DevOps — includes the Eclipse IDE, continuous delivery tools and availability monitoring
11.Application services — includes Blockchain, Message hub and business rules, among others
12.Integration — includes tools for building virtual bridges for hybrid cloud and multi-cloud environments, such as API Connect and Secure Gateway
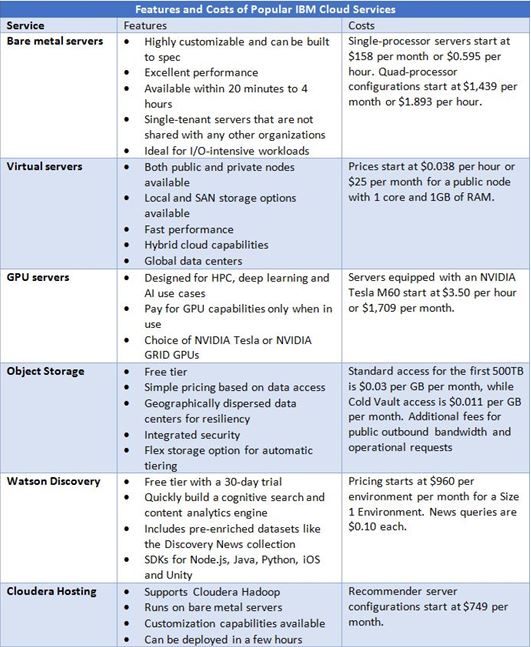
WHY YOU CHOOSE IBM CLOUD-
The IBM Cloud has a few unique offerings that its competitors can't match. For example, it is the only major cloud vendor that emphasizes its bare metal servers, which can be very attractive for organizations that have particular performance or security requirements. IBM also gives organizations a lot of flexibility and customization options that the other vendors don't have. On the downside, it can take up to several hours to configure and deploy a custom server, so these options don't offer the same speed as AWS, Azure or Google.
IBM also stands out in some other areas, like its cutting-edge blockchain offering, and its Watson cognitive computing capabilities. Ever since its Watson technology appeared on the television game show Jeopardy, IBM has been garnering attention for its artificial intelligence capabilities — although it does face stiff competition from Google and others.
Organizations that already use IBM technology, such as Power8 servers or IBM software, in their data centers will likely be drawn to the IBM Cloud in the same way that Microsoft users are drawn to Azure. The company has made significant investment in enabling hybrid cloud computing, and many of its cloud management and security tools work across hybrid and multi-cloud environments.
Gartner has noted, "Customers report positive experiences of IBM's Bluemix support and their business relationship. Strategic support of the hybrid deployment model and the broad spectrum of platform choices, from hosted to cloud-native, are well-suited to the variety of cloud migration strategies used by IBM customers." Similarly, in a survey conducted by Cowen & Co., IBM scored high for the quality of its IT support, but those surveyed cited its cost as a weakness.
LINK TO GO DEPTH AND SEARCH ABOUT IT-https://www.ibm.com/cloud/
FOURTH COMES-
GOOGLE CLOUD PLATFORM (GCP)-
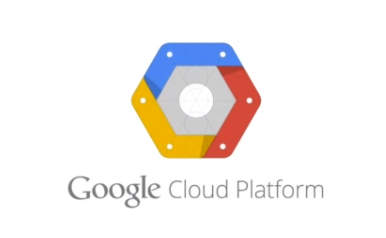
Google doesn't break out its cloud computing numbers, so it's very difficult to tell how much revenue its Cloud Platform generates. In a recent report, Synergy estimated that Google is fourth in the IaaS and PaaS market with a 4 percent share of the market. The report also noted that Google's cloud revenue is climbing rapidly, surging 108 percent year-over-year in 2015.
Like Amazon and Microsoft, Google offers a very full range of IaaS and PaaS services that span compute, storage, networking, big data, machine learning, developer tools and security. Some of its best-known cloud offerings include Compute Engine, App Engine, Container Engine, Cloud Storage and BigQuery.
Along with strong IaaS capabilities, it is Google's PaaS that really shines — and the Google Cloud Platform excels at big data, analytics and machine learning as well.
As the world's undisputed leader in Internet search, Google has undeniable expertise in running data centers. After Amazon launched its cloud computing service in 2006, Google put that data center expertise to work by launching a cloud service of its own. Soon it became known as one of the "big three" public cloud vendors, along with AWS and Microsoft.
Depending on which analyst report you read, today Google is either the third or the fourth largest public cloud vendor, behind Amazon, Microsoft and (possibly) IBM. While its larger competitors tout their broad portfolios of services, Google has a narrower focus and specializes in meeting the needs of developers. Through its Web search and advertising businesses, the company has become particularly adept at handling big data, and it has transferred those capabilities to its cloud computing business as well, developing a solid reputation for prowess in analytics, artificial intelligence and machine learning.
Google Cloud Platform Services
Google Cloud Platform's portfolio of cloud services isn't as extensive as Amazon's or Microsoft Azure's, but it offers some specialized tools for developers that are hard to find elsewhere. It organizes its cloud services into the following nine categories:
1.Compute — includes Google's original cloud service, the App Engine PaaS, as well as Compute Engine, Container Engine, Container Registry and the Cloud Functions serverless computing offering
2.Storage and Database — includes Cloud Storage object storage, Cloud SQL with MySQL or PostgreSQL database options, Cloud Bigtable NoSQL database, Cloud Spanner relational database, Cloud Datastore NoSQL database and Persistent Disk block storage
3.Networking — includes Virtual Private Cloud (VPC), Cloud Load Balancing, Cloud CDN content delivery, Cloud Interconnect for enterprise-grade connections to Google's Cloud and Cloud DNS for Web domain serving
4.Big Data — includes the BigQuery large-scale data warehouse, Cloud Dataflow for batch and stream data processing, Cloud DataProc with Spark and Hadoop capabilities, Cloud Datalab for analytics and visualizations, Cloud Dataprep for data preparation, Cloud Pub/Sub messaging, Genomics for genetic scientists and Google Data Studio for business reporting
5.Internet of Things — includes Google's Cloud IoT Core for secure device connection and management
6.Machine Learning — includes Google's Cloud Machine Learning Engine, as well as its APIs for job search, natural language, speech, translation, vision and video intelligence
7.Identity and Security — includes Cloud IAM identity and access management, Cloud Identity-Aware Proxy, Cloud Data Loss Prevention API, Security Key Enforcement, Cloud Key Management Service, Cloud Resource Manager and the Cloud Security Scanner
8.Management Tools — includes Stackdriver Overview, Monitoring and Logging, all of which support both Google Cloud Platform and AWS. This category also includes app optimization and deployment tools like Trace, Debugger, Cloud Deployment Manager, Cloud Endpoints, Cloud Console, Cloud Shell, Cloud Mobile App, Cloud Billing API and other Cloud APIs
9.Developer Tools — Includes the Cloud SDK with its command line interface for Google Cloud Platform services, container tools, Cloud Source Repositories, Cloud Tools for Android Studio, Cloud Tools for IntelliJ, Cloud Tools for Powershell, Cloud Tools for Visual Studio, Cloud Tools for Eclipse, Gradle App Engine Plugin, Maven App Engine Plugin and the Cloud Test Lab for On-Demand Testing
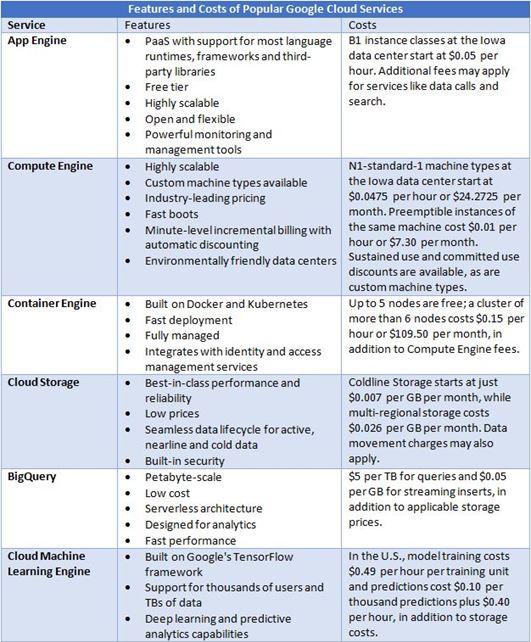
WHY YOU SWITH TO GOOGLE CLOUD PLATFORM-
Google's biggest advantage when it comes to cloud computing is its experience as the world's leading search engine. By powering Internet search, Google learned how to design and manage Web-scale data centers, and that expertise allows it to achieve high reliability and fast performance, as well as economies of scale that enable very low prices.
Those low prices are a key differentiator for Google. It has committed itself to price leadership in the public cloud, and offers a variety of discount programs and rightsizing recommendations to help customers keep costs low.
In addition, Google employs a vast number of developers itself, and that gives the company insight into what developers need and want from a cloud computing provider. Many of its cloud offerings are tailored for the needs of developers, particularly developers who are building cloud-native apps.
The company also touts its commitment to innovation and openness. It is a leader in the fast-growing area of data analytics and machine learning. It also boasts fast performance and easy set-up and deployment.
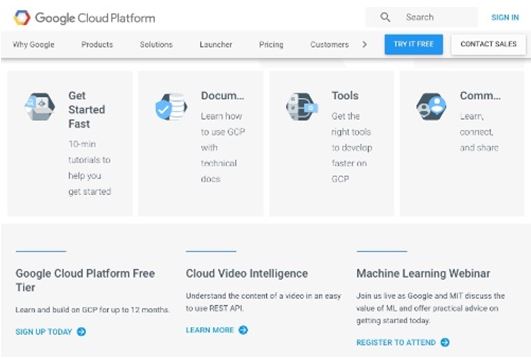
The Google Cloud Platform offers a diverse array of tools, and is considered particularly strong in data analytics.
LINK TO SEARCH MORE AND GO INTO DEPTH-https://cloud.google.com/